Control Structure
Selection Statements
A selection statement provides the means of choosing between two or more paths of execution. In React.js, selection statements are primarily used to make decisions and conditionally render components or elements based on certain conditions. React.js typically use both two-way and multiple-way selectors for handling different conditions, depending how many options or paths there need to manage in the application:
-
Two-way selectors
Used when there are only two possible conditions to handle, such as if statement and ternary operators
-
If-else statement
You can use traditional if statements to determine which component to render based on a condition. This method is straightforward and works well for more complex conditions and is often clearer when dealing with multiple lines of code.
Format:

Sample Code in our Sample Application:
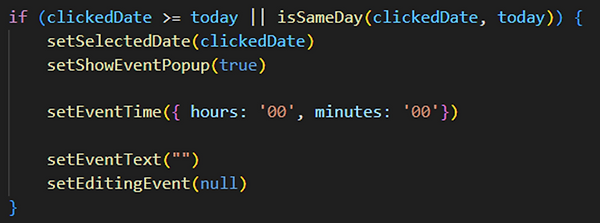
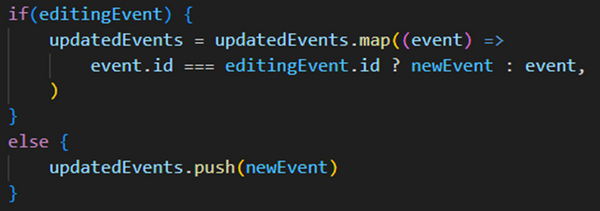

These code above show the if-else statements that we have used in our sample application.
-
Ternary operators
The ternary operator provides a concise way to conditionally render components inline between two components or outputs.
Format:

-
Multiple-way selectors
Used when there are more than two conditions to handle, such as if statement and ternary operators
-
Switch statement
Useful for rendering different components based on various conditions.
Format:

-
​Logical AND (‘&&’) operators
The logical AND operator can be used to render a component only if a certain condition is true. If the condition evaluates to false, nothing will be rendered.
Format:

This flexibility allows developers to create dynamic user interfaces that respond appropriately to different application states and user inputs.
Iterative Statements
An iterative statement is the repeated execution of a statement or compound statement is accomplished either by iteration or recursion. JavaScript with React framework does not introduce new iterative statements but relies on JavaScript’s native iteration methods to handle looping and repetition within components. There are two categories of iterative statements in React.js, which are counter-controlled loops and logically-controlled loops.
-
Counter-controlled Loops:
These loops are typically that increments or decrements with each iteration until a specific condition is met. Example:
-
for Loop
-
A for loop repeats until a specified condition evaluates to false.
Format:

-
The initializing expression initialization, if any, is executed. This expression usually initializes one or more loop counters, but the syntax allows an expression of any degree of complexity. This expression can also declare variables.
-
The condition expression is evaluated. If the value of the condition is true, the loop statements execute. Otherwise, the for loop terminates. (If the condition expression is omitted entirely, the condition is assumed to be true.)
-
The statement executes.
-
If present, the update expression afterthought is executed.
-
Control returns to Step 2 (Loops and Iteration - JavaScript | MDN, n.d.).
-
for…of Loop (iteratives over property values)
-
The statement creates a loop iterating over iterable objects (including Array, Map, Set, arguments object and so on), invoking a custom iteration hook with statements to be executed for the value of each distinct property (Loops and Iteration - JavaScript | MDN, n.d.).
-
Format:

-
for…in Loop (iterates over property name)
-
The statement iterates a specified variable over all the enumerable properties of an object (Loops and Iteration - JavaScript | MDN, n.d.).
-
Format:

-
Logically-controlled Loops:
These loops are controlled by a condition that must be true for the loop to continue. They do not rely on a counter but rather on logical conditions (True or False).
-
while Loop
-
The condition test occurs before statement in the loop is executed.
-
If the condition returns true, statement is executed and the condition is tested again. If the condition returns false, execution stops, and control is passed to the statement following while (Loops and Iteration - JavaScript | MDN, n.d.).
Format:

-
do…while Loop
-
Statement is always executed once before the condition is checked.
-
Repeats until a specified condition evaluates to false (Loops and Iteration - JavaScript | MDN, n.d.).
Format:

User-Located Loop Control Mechanisms
User-located loop control mechanisms are the methods or abstractions provided by objects to iterate over their elements. In React.js, user-located loop control mechanisms primarily refer to the methods and techniques used to manage iterations within components. These mechanisms allow developers to control the flow of rendering lists and handling data dynamically. Here are the user-located loop control mechanisms that have used in React.js:
-
for…each Loop:
The loop executes a provided callback function once for each element in an array. It is typically used for performing side effects like logging or modifying external variables but does not return a new array (The Ultimate Guide to React Iterate Over Object, n.d.).​
Format:

-
map method:
The map method creates a new array by calling a provided function on every element in the original array. It is typically used to transform the elements of an array (The Ultimate Guide to React Iterate Over Object, n.d.).
Format:

Sample Code in our Sample Application:

This line of code shows the mapping over arrays (method) which iterates over days and weeks to render calendar elements.
-
labelled statement:
-
A label provides a statement with an identifier that can be referred to it elsewhere in the program.
-
Used to identify a loop, and then use the break or continue statements to indicate whether a program should interrupt the loop or continue its execution (Loops and Iteration - JavaScript | MDN, n.d.).
Format:

-
continue statement:
-
Can be used to restart a while, do-while, for, or label statement.
-
When continue be used without a label, it terminates the current iteration of the innermost enclosing while, do-while, or for statement and continues execution of the loop with the next iteration. In contrast to the break statement, continue does not terminate the execution of the loop entirely. In a while loop, it jumps back to the condition. In a for loop, it jumps to the increment-expression.
-
When you use continue with a label, it applies to the looping statement identified with that label (Loops and Iteration - JavaScript | MDN, n.d.).
Format:
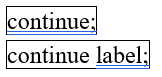
-
break statement:
-
Used to terminate a switch or loop, or in conjunction with a labelled statement.
-
When break be used without a label, it terminates the innermost enclosing while, do-while, for, or switch immediately and transfers control to the following statement.
-
When you use break with a label, it terminates the specified labelled statement (Loops and Iteration - JavaScript | MDN, n.d.).
Format:

Iteration Based on Data Structure
In React.js, iteration based on data structure refers to rendering components or elements by looping over data structures (like arrays, objects, or maps). This approach is commonly used to dynamically generate lists, tables, menus, or any repetitive UI components. Here’s an overview of how iteration works with different data structures in React:
-
Arrays
​Arrays are commonly iterated using methods like map, forEach, and filter. The map method is particularly useful as it creates a new array populated with the results of calling a provided function on every element in the calling array.
Sample Code in our Sample Application:

Iterative based on data structure includes iterating over arrays or objects using methods like map, forEach, etc. In the provided code, we have map function used to iterate over events.
-
Objects:
When it comes to objects, since they do not have built-in iteration methods like arrays, developers often convert objects into iterable formats using methods such as Object.keys(), Object.values(), or Object.entries().
-
Object.keys(): Returns an array of a given object's own enumerable property names.
-
Object.values(): Returns an array of a given object's own enumerable property values.
-
Object.entries(): Returns an array of a given object's own enumerable string-keyed property [key, value] pairs.