
Arithmetic Expressions

Operators
Arithmetic evaluation was one of the motivations for the development of the first programming languages. These expressions consist of operators, operands, parentheses, and function calls. In React.js, arithmetic expressions are used to perform calculations and manipulate numerical values.
Examples:
1. Addition
Use the operator ‘+’ to add two or more numbers.

2. Subtraction
Use the ‘-’operator to subtract one number from another.

3. Multiplication
Use ‘*’ operator to multiply two or more numbers.

4. Division
Use / operator to divide one number by another.

5. Modulos
Use the ‘%’ operator to get the remember of a division operation.

6. Parentheses
Use parentheses to group arithmetic expressions and control the order of operations.

JavaScript maintains the mathematical order of operations, also called PEMDAS - Parentheses, Exponents, Multiplication, Division, and Addition and Subtraction. This means that calculations inside the parentheses are done first, followed by exponents, then multiplication and division from left to right, and finally addition and subtraction from left to right.
​
Therefore, arithmetic expressions are an asset in JavaScript programming commonly used for mathematical computation and numerical value manipulation. So, if you want to learn how to write proper JavaScript that will perform some complex calculations you should sort out different arithmetic operators as well as the order of operations (Bansikah, 2023).
Sample Code for React.js in our sample application:
-
currentMonth + 1 is an example of using the addition operator to calculate the days in the next month.

Operator Precedence Rules
Operator precedence describes the order in which operations are performed in an arithmetic expression. The typical precedence levels are starting with parentheses, unary operators such as multiplication and division that have higher precedence than addition and subtraction. Operator precedence will be handles recursively. So, in JavaScript, as in traditional mathematics, multiplication is done first (Operator Precedence in JavaScript, 2024).
Examples:

Then, when using parentheses, operations inside parentheses are usually computes first:

Operations with the same precedence like multiplication and division in one mathematic equation are computed from left to right:

Basically, parentheses content is evaluated first in the expression in rates of mental calculation. Just like how the function are executed before the result is used in the rest of the expression.
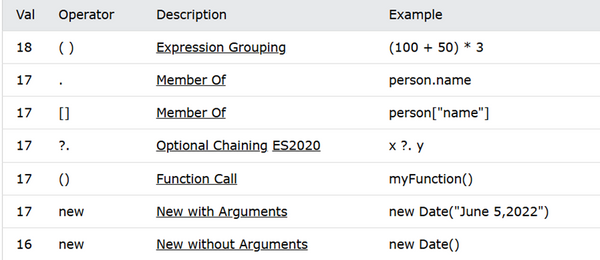
Postfix increments are executed before prefix increments.
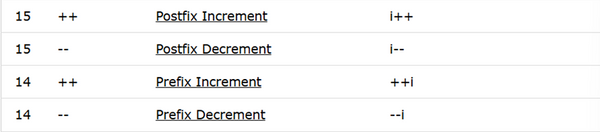
The same rules apply to postfix unary operators, that is, (++) and (- -). Fortunately, both have a higher precedence than any binary operator and are. Therefore, grouped as you would expect them to be. However, ++ is a value returning prefix operator and not a reference so you cannot link together multiple increments as is done in C.
Not operators:

Unary operators:
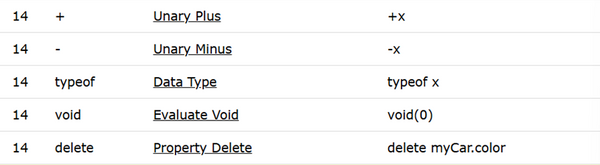
Arithmetic operators, exponentiations are executed before multiplications. So, exponentiations are a high precedence than multiplication.
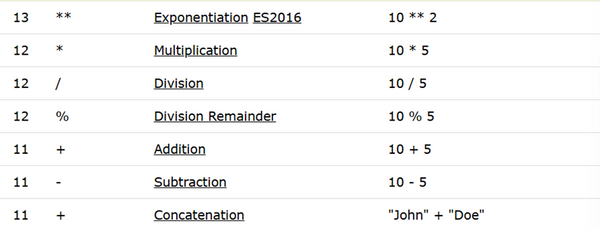
Relational operator is always evaluated first, resulting in false. Then, the logical evaluated, but since one of its operands is false, the result is also false.
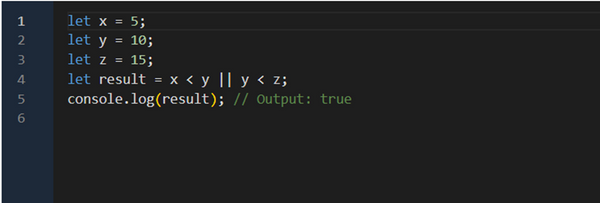
For example, ‘<’ is less than truth that is equal to true. Next comes the logical OR logical operator ‘||’, and as one of the arguments is true, the results is true also. However, it is important to remember that understanding operator precedence is crucial. However, if utilized inappropriately, it may result to development of compliances. Of course, wherever there is doubt as to the order in which the operations are performed, it is best to use parentheses.
Logical Operators:

Sample Code for React.js in our sample application:
-
The + operator has higher precedence than the === operator, ensuring day + 1 is evaluated first.
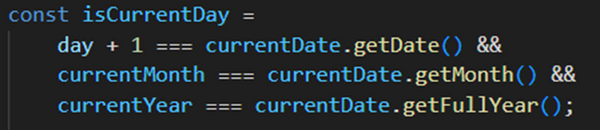
Operator Associativity Rules
Associativity rules specify the order in which operators with the same precedence are evaluated. There are two types of associativity: left-to-right and right-to-left. Left-to-right associativity means that operators with the same precedence are evaluated from left to right.
For example, the addition operator ‘+’ has left-to-right associativity, so expressions like 3+4+5 are evaluated as (3+4) + 5 and result in 12. Right-to-left associativity means that operators with the same precedence are evaluated from right to left.
Another example to explain, the assignment operator ‘=’ has to be right-to-left associativity, so expressions like a=b=c are evaluated as a a= (b=c) and result in c being assigned to both b and a (W3Schools.com, n.d.-b).
In this example code of React.js, the subtraction operator ‘-‘ has left-to-right associativity. The expression is evaluated as (3-2)-1 which result in 0
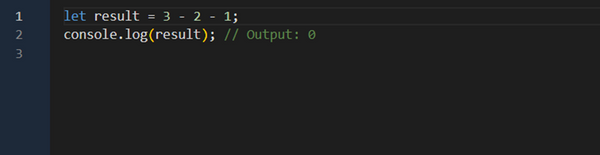
In this example code of React.js, the compound assignment operator += has right-to-left associativity. The expression is evaluated as a += (b += c), which results in a being assigned the value of 6, b being assigned the value of 5, and of retaining its original value of 3.
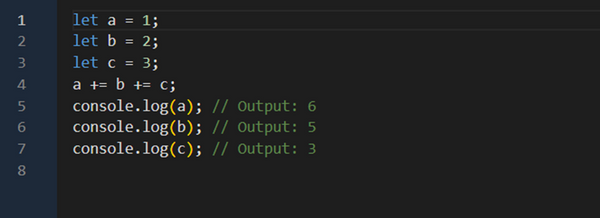
Altogether, operator precedence and associativity rules should be learnt in order to programme correct and efficient code in JavaScript. These rules define the order in which the operators are applied or evaluated in the given expressions and knowing such rules can help to have a big effect on the answer of any expressions involving many operations. By applying using parentheses and understanding rules of operator precedence several directives of associativity, one can easily write clear code.
Sample Code for React.js in our sample application:
-
The addition operator + is left-associative, so calculations like day + 1 are evaluated from left to right.

Operand Evaluation Order
The operand evaluation order contains with variables fetching the value from memory. Then, the constants sometimes fetch from the memory; sometimes the constant is in the machine language instruction. Parenthesized expressions evaluate all operands and operators first. In JavaScript, the operands to operators are evaluated from left to right. The left operand of a binary operator is fully evaluated before any part of the right operand is evaluated.
For example, if the left operand contains an imperative that assigns a value to a variable and the right operand contains a constant or other expression that includes reference to the variable, then an evaluation for the operation will yield the value with the left operation mark since it came first (Expressions, n.d).
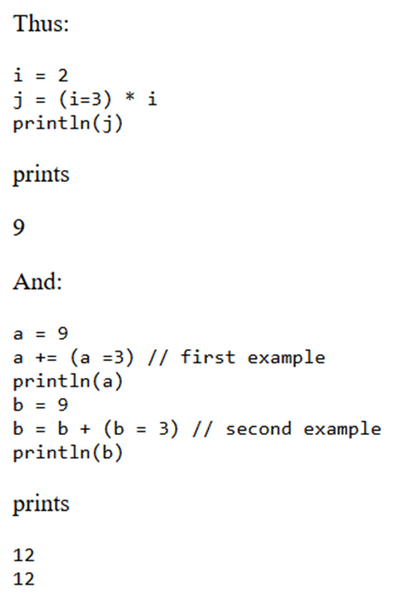
Every operand of an operator (except for &&, ||, and ? :) is fully examined before any part of the operation itself is undertaken. Within a function or constructor call value or objects the arguments expressions are placed in the parentheses if any separated by commas. The evaluation each argument expression does not proceed past any point before any phase of any argument expression to the right of that argument expression completes.
Sample Code for React.js in our sample application:
-
The logical || operator ensures left-to-right evaluation, stopping if the first condition is true.
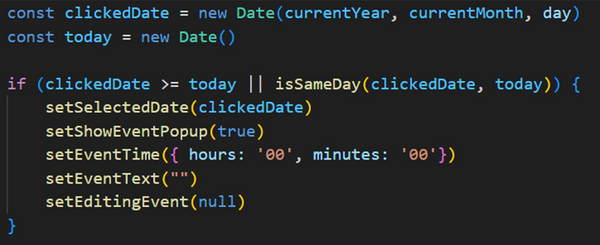
Functional Side Effects
Functional side effects happen when a function changes either one of its parameters or global variables. In React.js, a side effect stands for any operation or action that happens within a component function, often after rendering, but not related to rendering the graphical user interface. Side effects include tasks such as (Upreti, 2024):
-
Data Fetching: Sending out requests at different times to gather data for an API or a server.
-
State Updates: Changing the state that propels re-rendering, for example with new context, new Redux state, or a new local component state.
-
DOM Manipulation: That manipulate the DOM such as changing the title of a web page or adding or removing classes.
-
Subscriptions: Registering for various external data feeds or event notifications that is receiving subscription if that is how it is defined.
React.js components are supposed to mainly render UI based on its state and/or props, with uses the paradigm of Functional Programming. But many applications involve relations with the outside world, say, to retrieve data or respond to certain user’s events are all side effects. If these side effects are handled appropriately, React.js components stay comprehensible, performant, and easy to sustain.
​​
​
React comes equipped with builtin way to handle side effects in functional components in the form of the useEffect hook. Here’s how useEffect works:
-
Syntax: The useEffect hook is called passing as its effect function the client component, and as the dependency array an empty array that tells React that this is an optional update.
​
-
Effect Function: Function that includes the code of the side effect – such as fetching data or changing the DOM.
​
-
Dependency Array: An optional array that lists values, on which the Effect depends. The effect runs:
-
After every render if the array is empty ([ ]).
-
If any of the dependencies occur.
-
None Of Them However, if omitted, it is rendered only once after the first call.
-
Sample Code for React.js in our sample application:
-
The setCurrentMonth and setCurrentYear calls have side effects of changing the state values.

Referential Transparency
A program has the property of referential transparency if any two expressions in the program that have the same value can be substituted for one another anywhere in the program, without affecting the action of the program. A referential transparency can help a team in the following ways. Semantics of a program is much easier to even know if it has referential transparency.
Since programs in them do not have variables, programs written in assembly language are not easily understood. The referential pure functional languages are transparent. State means that a given function has some data that is retained within the system, and this is forbidden stored in local variables. The dependencies of a function, hence, must be an outside value if the function so requires it constant (there are no such variables), it is tangible, quantifiable, measurable. So, the value of a function depends only on its parameters. Referential transparency is an advantage.
A drastic change of semantics of a program is much easier to information to provide an adequate answer to the complex research question, each sub-research question should understand if it has referential transparency. This results from the fact that programs don’t have variables, in only referential pure functional languages transparent. Functions cannot have state which would be stored in local variables If a function uses an outside value, the relation must be the variable’s only job. constant (it means there is no variation). So, the value whether a function is defined or not depends only on its parameters (Smiley, 2022).
This means that call by reference is meaningful if and only if the function is a pure function that has parameters which are referentially transparent. To achieve this in JavaScript we use immutable-js to create immutable data, and closures to ensure you cannot measure our object and change state. Here follows another class-based approach using closures and a weak map to shelter private members:
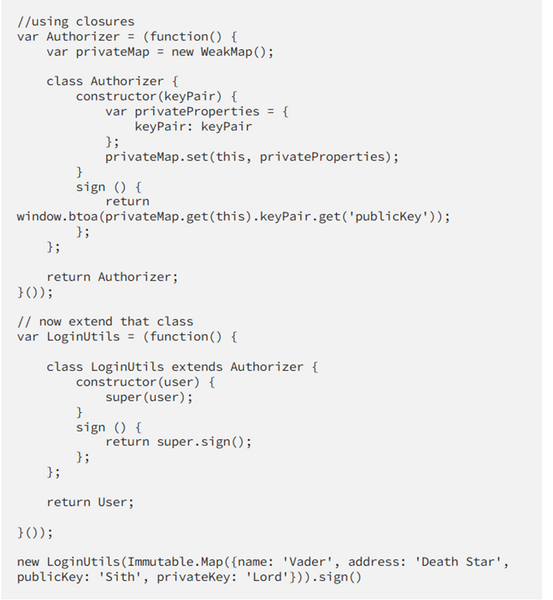
Enclosing the class in a self-executing function also protects the privateMap variable which exists only inside the function call from other observers. At first, it can be thought that the Authorizer.sign method is referentially transparent. This means that it can be done using memoization. The use of memoization can boost the efficiency of your web as well as your mobile applications.
Sample Code for React.js in our sample application:
-
The isSameDay function is referentially transparent because it depends only on its input arguments without side effects.
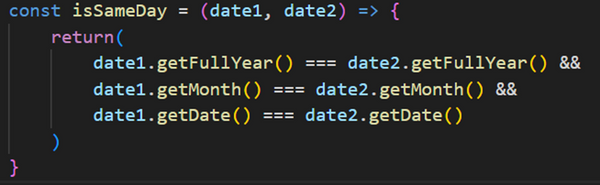