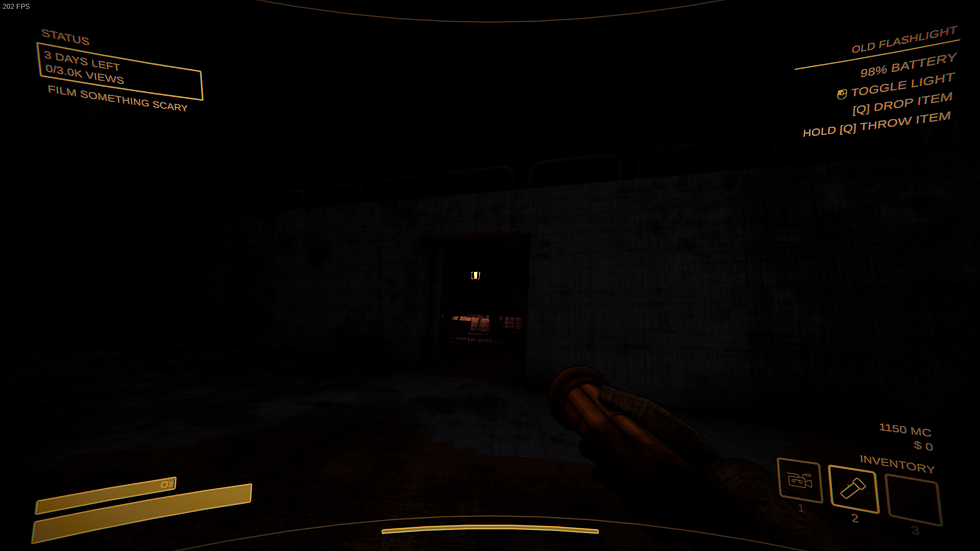
Name
Naming Convention for Variables
-
The variable name of React.js can start with any letters, underscore (_) and dollar sign ($), and be followed by any letters, dollar sign, underscore, and numbers WITHOUT ANY SPACING (JavaScript Style Guide, n.d.)
​
Below are the sample codes that show the valid variable name declaration:
Variable name declaration
1. currentDate
A variable that holds the current date as a Date object.

2. currentMonth
A state variable that stores the current month (as a number, 0-11).

3. currentYear
A state variable that stores the current year.

4. selectedDate
A state variable that holds the date selected in the calendar.

5. showEventPopup
A boolean state variable to track whether the event popup is visible.

6. events
A state variable that stores an array of event objects.

7. eventTime
A state variable that stores the time (hours and minutes) for an event.

8. eventText
A state variable that holds the text of an event.

9. editingEvent
A state variable that stores the event being edited (if any).

10. daysInMonth
A variable that calculates the number of days in the current month.

11. firstDayOfMonth
A variable that gets the day of the week for the first day of the current month.

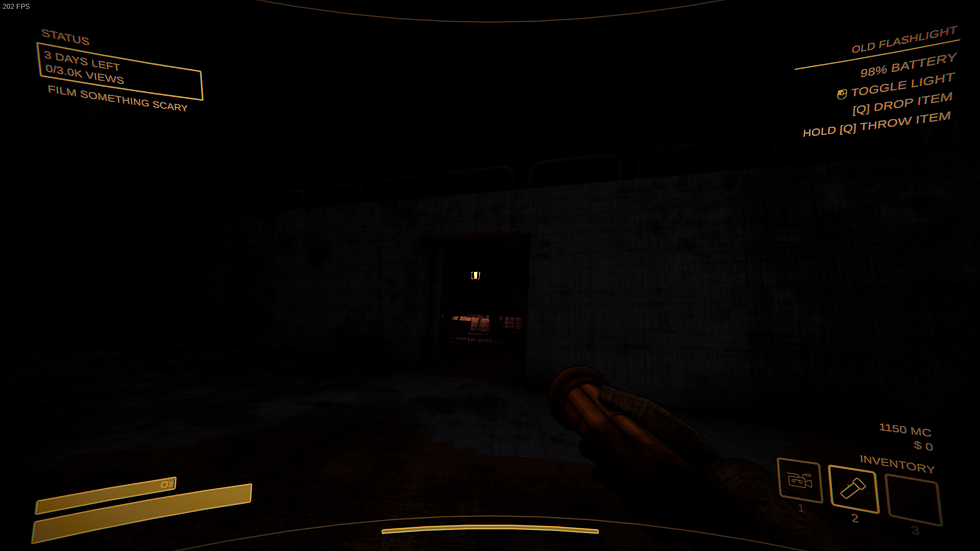
Array name declaration
1. daysOfWeek
An array containing the days of the week.

2. monthsOfYear
An array that contains the months of the year.

3. updatedEvents (temporary updated events list)
A temporary array used for sorting or modifying the event list.

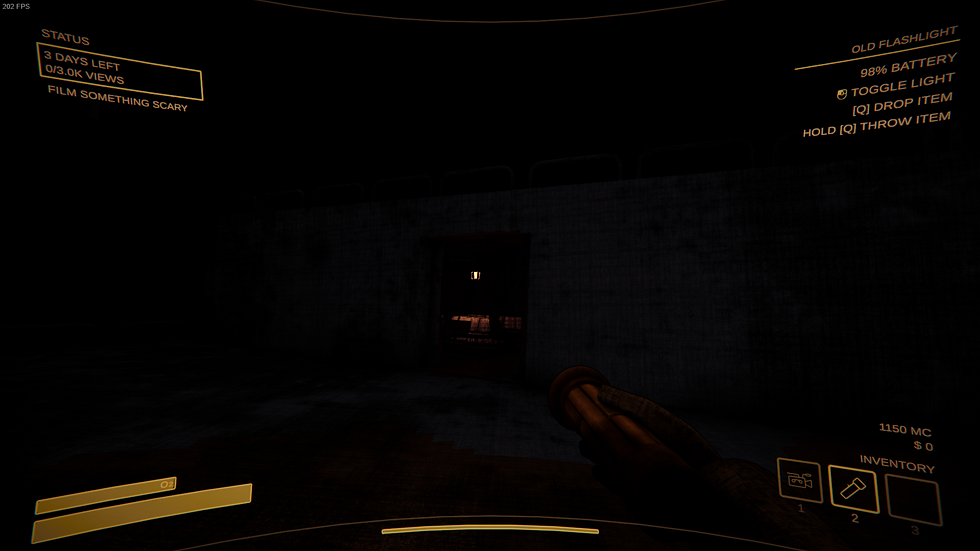
Function name declaration
1. CalendarApp
The main functional component that renders the calendar app.

2. prevMonth
A function that decrements the current month and adjusts the year if necessary.

3. nextMonth
A function that increments the current month and adjusts the year if necessary.

4. handleDayClick
A function that handles user clicks on calendar days to show the event popup.

5. isSameDay
A utility function that checks if two dates are the same.
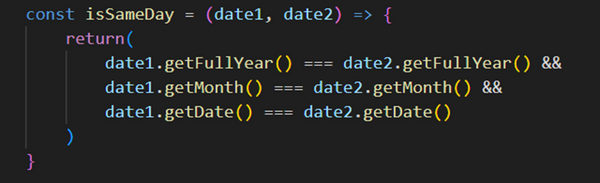
6. handleEventSubmit
A function that handles the submission of an event to add or update it in the event lists.

7. handleEditEvent
A function that allows users to edit an existing event.
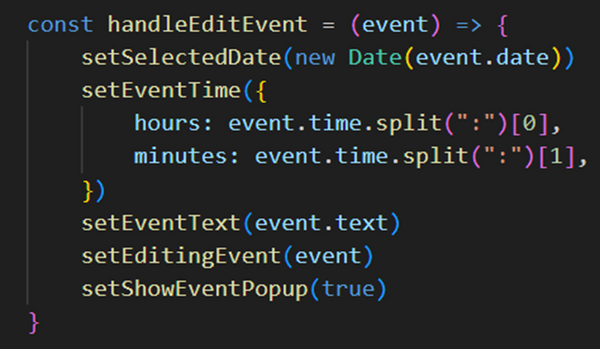
8. handleDeleteEvent
A function that deletes an event based on its ID.

9. handleTimeChange
A function that updates the event time (hours and minutes) based on the user input.

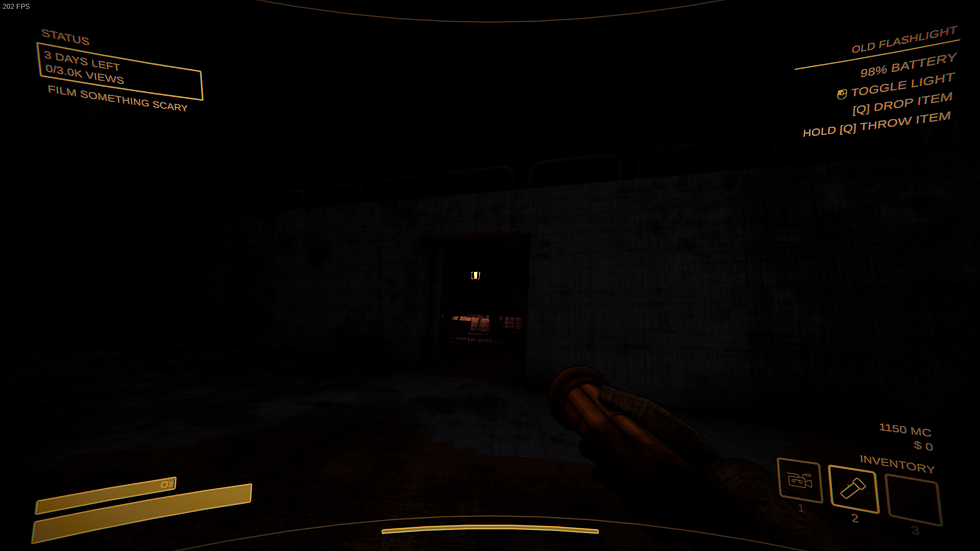
Method name declaration
JavaScript Methods
1. new Date() (creates a new date object)
A JavaScript method that creates a new Date object, representing the current date and time.

2. .getMonth()
A JavaScript method that returns the month (0–11) from a Date object.

3. .getFullYear()
A JavaScript method that returns the full year (e.g., 2024) from a Date object.

4. .getDate()
A JavaScript method that returns the day of the month (1–31) from a Date object.

5. .getDay()
A JavaScript method that returns the day of the week (0–6) for a given date (e.g., Sunday = 0).

6. .push() (adds an element to an array)
A JavaScript method that adds one or more elements to the end of an array.

7. .map() (iterates over an array)
A JavaScript method that creates a new array populated with the results of calling a function on every element in the array.

8. .filter() (creates a new array based on a condition)
A JavaScript method that creates a new array with all elements that pass a test implemented by the provided function.

9. .sort() (sorts an array)
A JavaScript method that sorts the elements of an array in place. In this case, it sorts the events chronologically by date.

React Methods
1. useState()
A React hook that lets you add state to functional components. It returns the current state and a function to update it.

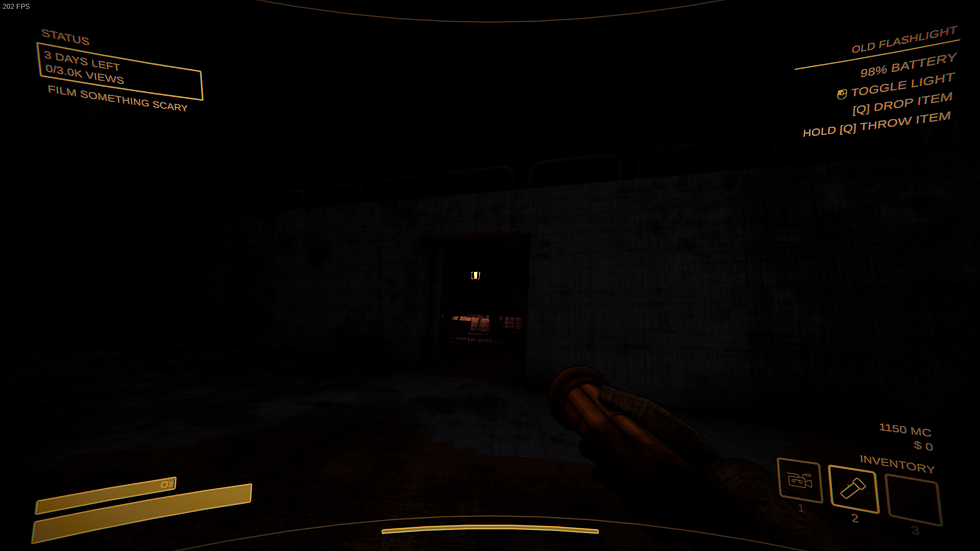
Boolean variable name declaration
1. showEventPopup (tracks if the event popup is visible)
A boolean state variable that toggles the visibility of the event popup.

2. editingEvent (tracks if an event is being edited)
A boolean-like state variable (nullable) that checks whether an event is being edited.

3. isCurrentDay (used to highlight the current day in the calendar)
A boolean variable that checks if the calendar day is the current day.

4. hasEvent (checks if a day has an event)
A boolean variable that checks if the calendar day has an event.

5. Conditionals like clickedDate >= today or isSameDay(clickedDate, today) return booleans.
clickedDate >= today:
A condition that checks if the clicked date is today or in the future.

isSameDay (clickedDate, today):
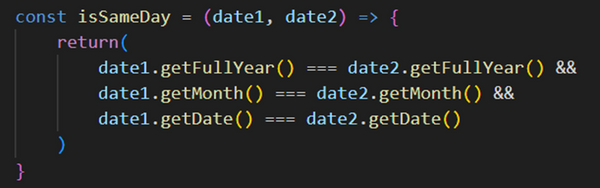
A boolean condition that checks if clickedDate and today are the same date. The isSameDay function compares both dates for equality.
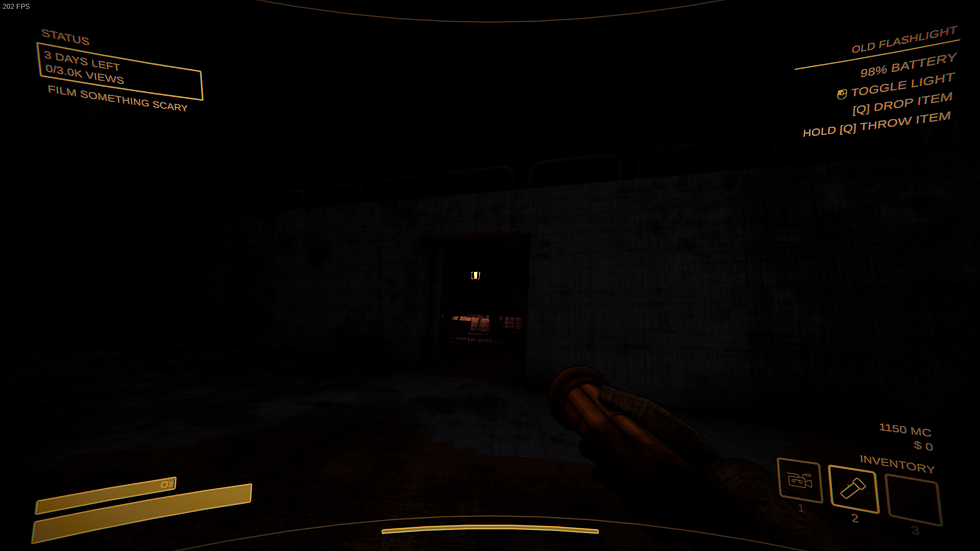
-
JavaScript variable names are case-sensitive. Lowercase and uppercase letters are distinct. The most recommended way to declare JavaScript variables is with camel case variable names to ensure that there aren’t multiple variables with the same name. The names of variables should be self-explanatory and describe the stored value (10 JavaScript Naming Conventions Every Developer Should Know, n.d.)
-
Meanwhile, the length of variable names in React.js, or JavaScript in general, is not fixed; it can vary depending on the developer's choice.
-
A name cannot be a keyword, contain spaces, or start with a special character. This is because they are be used by the language itself. Below are the list of the reserved words that cannot be used as variables, labels, or function names(JavaScript Reserved Words, n.d.):
abstract
byte
const
do
export
float
implements
interface
null
return
synchronized
true
volatile
Date
encodeURI
Number
undefined
anchors
checkbox
constructor
elements
escape
form
innerWidth
navigate
history
opener
pageXOffset
password
radio
secure
status
top
arguments
case
continue
double
extends
for
import
let
package
short
this
try
while
hasOwnProperty
length
Object
valueOf
area
clearInterval
clearTimeout
clientInformation
isPrototypeOf
encodeURIComponent
layers
navigator
image
option
pageYOffset
pkcs11
reset
select
submit
unescape
await
catch
debugger
else
false
function
in
long
private
static
throw
typeof
with
Infinity
Math
prototype
alert
assign
close
crypto
element
event
forms
link
frames
images
outerHeight
parent
plugin
screenX
self
taint
untaint
boolean
char
default
enum
final
goto
instanceof
native
protected
super
throws
var
yield
isFinite
NaN
String
all
blur
closed
decodeURI
embed
fileUpload
frame
location
frameRate
offscreenBuffering
outerWidth
parseFloat
prompt
screenY
setInterval
text
uwindowntaint
break
class
delete
eval
finally
if
int
new
public
switch
transient
void
Array
isNaN
name
toString
anchor
button
confirm
document
embeds
focus
innerHeight
mimeTypes
Heading 4
open
packages
Heading 4
propertyISEnum
scroll
setTimeOut
textarea
-
Reserved Words in React.js