
Procedures & Functions
Procedures
Procedures are one of the two categories of subprograms. Procedures are a collection of statements that define parameterized computations, and it does not return values. Instead, it provides a list of instructions that contains the activity of the actions or operations and can be anything that the programmer would want the procedure to do. A procedure is essentially a function with or without a return statement that has no value to return (Functions & Procedures in JavaScript | OCR a Level Computer Science Revision Notes 2017, 2024).
Sample Code in our sample application

This function is responsible for removing an event from the list of events when a user clicks the delete icon. It modifies the events state to remove the selected event.
Functions
Functions structurally resemble procedures but are semantically modeled on mathematical functions. It can also be defined as not returning values and used as procedures. Function is an algorithm that contains some statements, performing a specific mission and can be run from any points in the program. Functions can receive parameters (arguments) and they can give a single output value (Functions & Procedures in JavaScript | OCR a Level Computer Science Revision Notes 2017, 2024).
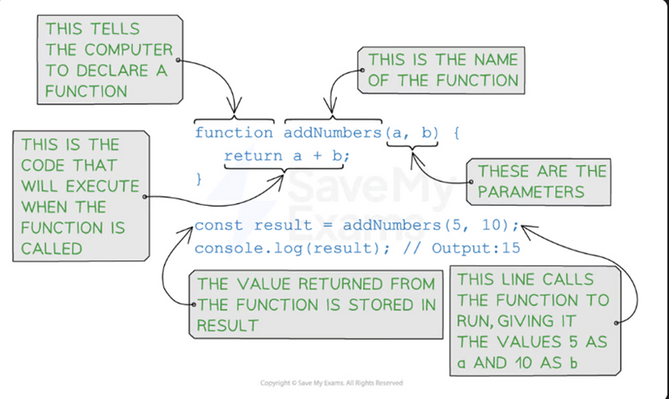
The picture above explains how to define a function.
The line function addNumbers(a , b) {…} tells the computer to announce a new function which is to be named addNumbers.
The keyword function is employed to declare the function, next, there is the name of the function addNumbers. Between the parentheses, the letters a and b are parameters of the function. Parameters are labels of arguments that the function is going to use at the time of its call. The code written next to the function’s header with the help of an equals sign (=) and located in the curly braces {return a + b; } is a function’s body. This is the function of the function that returns values when the functions are invoked.
In this case, the function simply takes two parameters a & b, and returns the sum of parameters of a and b. As for the notion of how the function is called, the example of using the line const result = addNumbers(5, 10); works perfectly. Here 5 and 10 are the arguments that accompany this function for the sake of a and b respectively.
The function is invoked with these values, and the result, which is 15, arrives at this after adding 5 with 10. The obtained value after the function call equals to 15 and its value is put in the result constant. The line console.log(result); which has the effect of writing the value of the variable result to the systems console – the output will be 15.
Example:

Sample Code in our sample application

prevMonth is a function that updates the month and year in the calendar.
Differences between procedures and functions
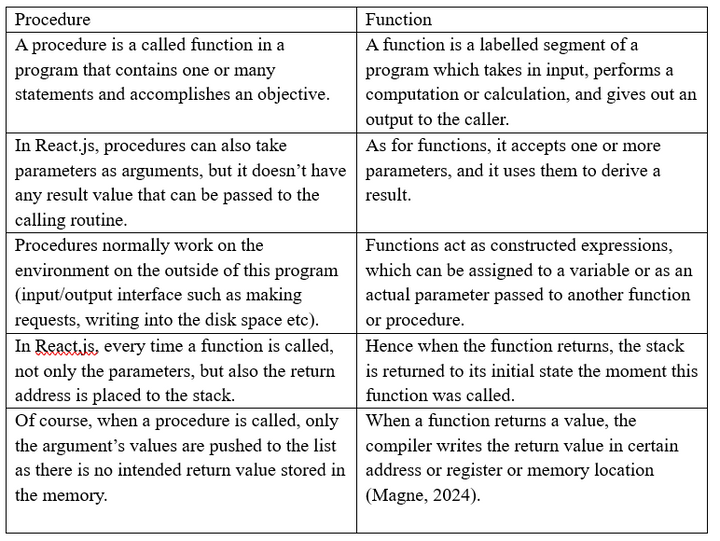