Assignment Statement
In React.js, assignment statements are used to assign values to variables, states, props, and other expressions.
-
General Syntax:
-
The general syntax of assigning the variable in React.js is let variableName = value;
-
-
Basic Assignment:
-
The assignment operator ‘=’ assigns a value from the right-hand side to a variable or property on the left-hand side.
-
-
Compound Assignment Operators:
-
React.js also includes various compound assignment operators that combine an operation with an assignment. In most cases, the syntax of expression: a op= b, where op is an operator, is equivalent to the expression: a = a op b (Assignment Expressions - JavaScript: The Definitive Guide, 6th Edition [Book], n.d.).
​The table below shows the list of compound assignments in React.js:
-
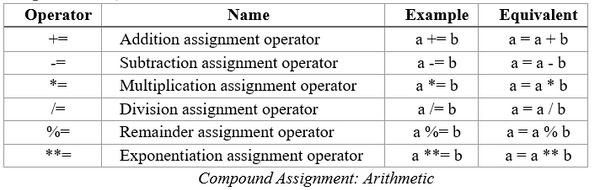
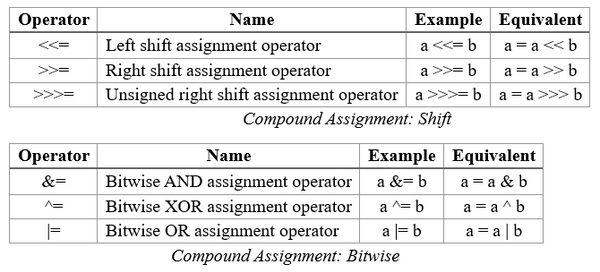
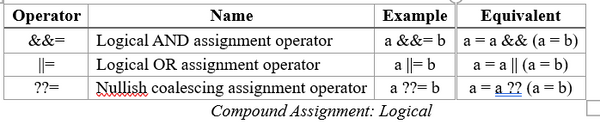
-
Unary Assignment Operators:
-
A unary operation is an operation with only one operand. This operand comes either before or after the operator. React.js does not specifically introduce unary assignment operators but utilizes JavaScript's existing operators. Unary operators can be used alongside React code but do not directly relate to how values are assigned in state or props management (How To Use JavaScript Unary Operators | DigitalOcean, n.d.)
-
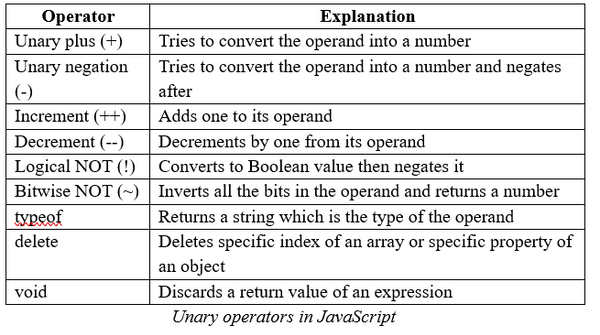
-
Multiple Assignment
-
Chaining Assignment
-
React.js allows chaining assignments, meaning you can assign the same value to multiple variables in one statement.​​
-
-
Destructuring Assignment
-
React.js allows for destructuring assignments, which can be particularly useful in React when dealing with state or props
-
-
-
Mixed-Mode Assignments
-
Mixed-Mode Assignment typically refers to assigning values of different types to variables or using various types in operations.
-
Mixed-mode assignment works in React.js because React relies on JavaScript’s dynamic typing. However, React itself does not have a concept of “Mixed-Mode Assignment” because it is a JavaScript library for building user interfaces. Thus, using such assignments should be done cautiously to avoid unexpected behaviour and bugs.
-
Recommended:
-
Use consistent data types when possible.
-
-