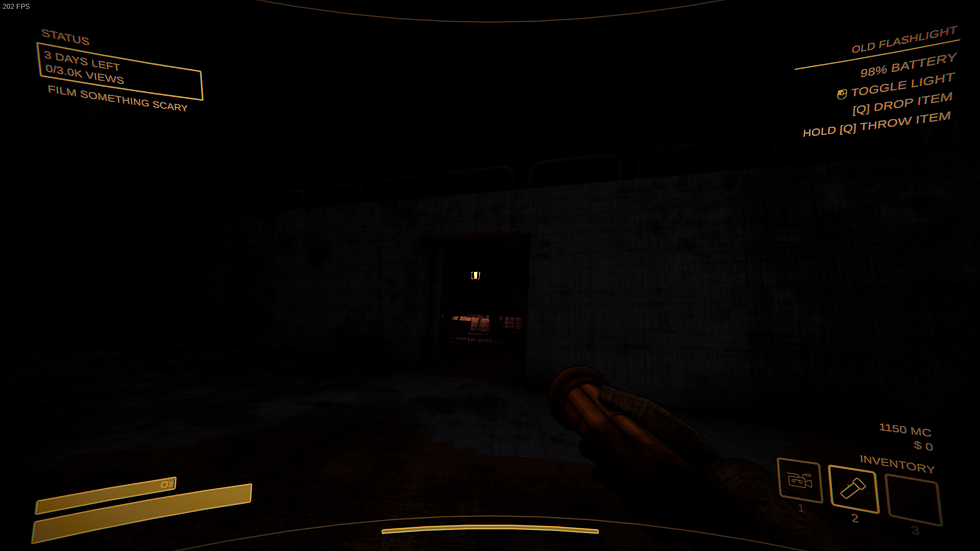
Lifetime
The lifetime of React.js revolves around the duration for which a variable is accessible and bound to a specific memory location. This can vary depending on where and how the variable is declared:
-
Global variables – throughout the lifetime of the application
-
Local variables – During the execution of the function or block in which they are declared
-
Block-scoped variables – During the block execution in which they are declared (typically inside {})
-
State variables in React components – As long as the component instance exists
-
Ref variables in React components – As long as the component instance exists
The lifetime of variables and components of React.js is managed through React’s lifecycle methods, reflecting a blend of these variable lifetime concepts. The component lifecycle of React.js which goes through from creation to removal from DOM is categorized into three phases:
-
Mounting: Putting elements into the DOM
-
constructor() – Initialize state and bind event handlers
-
static getDerivedStateFromProps() – Updates state from props (rarely used).
-
render() – Describes what the UI should look like.
-
componentDidMount() – Runs after the component is mounted, ideal for data fetching or DOM updates.
-
-
Updating: A component is updated whenever there is a change in the component’s state or props
-
static getDerivedStateFromProps() – Updates state before rendering.
-
shouldComponentUpdate() – Determines whether the component should re-render.
-
render() – Updates the UI based on new state or props.
-
getSnapshotBeforeUpdate() – Captures DOM state before updates.
-
componentDidUpdate() – Executes after the component re-renders, useful for side effects.
-
-
Unmounting: Component is removed form the DOM
-
componentWillUnmount() – Used to clean up subscriptions, timers, or other side effects (React Lifecycle, n.d.).
-
Dynamic Lifetime:
React.js’ lifetimes are vary based on user interactions, data changes, and other factors during the application’s runtime.
-
Components and their associated variables, state, and props are created and destroyed dynamically based on user interactions and routing.
-
When a component is mounted, its lifetime starts; when it is unmounted, its lifetime ends.
-
Example in our sample application:
The variables like currentMonth, selectedDate, and showEventPopup have a lifetime tied to the component's rendering cycle. For example, when the component re-renders (e.g., setCurrentMonth is called), these variables will be reset or updated.
Sample code in our sample application:
In React.js, variable lifetimes align with different storage binding categories:
-
Stack-Dynamic Binding (common in React) - Variables created when functions or components are called and destroyed when they return.
-
Explicit Heap-Dynamic Binding - Objects managed manually via references.
-
Implicit Heap-Dynamic Binding (very common) - Variables allocated when assigned values.
In our sample application code, the variables (like currentMonth, eventText, etc.) are not explicitly typed. This is because JavaScript is a dynamically typed language, meaning that variable types are determined at runtime. For instance, the currentMonth variable is initialized as a number based on the current date, specifically by calling currentDate.getMonth().
