
Scope
The scope of a variable in React.js is determined by where and how the variable is declared, modified or used. This could prevent unintended interactions and ensure that data is accessible only where it's meant to be (Scope and Closures in React and JavaScript - Filippo Rivolta - FrontEnd Developer, n.d.). React.js, being a JavaScript library, follows the same scoping rules as JavaScript. There are several of the different types of variable scope for React.js:
-
Global Scope – Accessible everywhere
-
Variables declared globally (outside any function or block) have global scope (more commonly known as global state)
-
All scripts and functions on a web page can be accessed it.
-
If the value that is assigned to a variable has not been declared, it will automatically become a global variable (JavaScript Scope, n.d.).
-
Global variables can be incredibly useful for storing application-wide data, their unrestricted accessibility can lead to naming conflicts and difficult-to-track bugs.
-
-
Function Scope – Accessible only within the function or any nested functions
-
Variables defined inside a function are not accessible (visible) from outside the function.
-
JavaScript has function scope: Each function creates a new scope.
-
-
Block Scope – Accessible only within the block
-
Variables declared inside a { } block cannot be accessed from outside the block
-
Variables declared with the var keyword can NOT have block scope.
-
-
Local/Component Scope – Accessible only within the component
-
Variables declared within a JavaScript function, are LOCAL to the function
-
Since local variables are only recognized inside their functions, variables with the same name can be used in different functions.
-
Local variables are created when a function starts and deleted when the function is completed.
-
They would be re-created on each render, potentially leading to performance overhead.
-
-
Module Scope – Accessible within the module unless exported
-
In React, each file is treated as an ES6 module, meaning the code written within the file is automatically in the module scope.
-
Accessible only within the module unless exported.
-
You can utilize the import and export statements to share and access the module scope in React.
-
The variables and functions defined within it are cached in JavaScript memory.
-
They are not recreated during component re-renders, providing a slight performance advantage (React: Scopes Demystified. Understanding the Different Contexts In… | by Jonatan Ramhöj | Medium, n.d.)
-
Scoping
React.js follows JavaScript’s scoping method, which is lexical (static) scoping. This means the scope of a variable is determined at compile time based on the structure of the code. Variables declared within a function are only accessible within that function and any nested functions, regardless of where the function is called.
Advantages of static scoping:
-
The code is easier to understand and predict the behaviour of the code
-
Reduces the risk of unintended access and side effects, making the code more secure and maintainable (Closures - JavaScript | MDN, n.d.).
Sample JavaScript Code of Static Scoping:
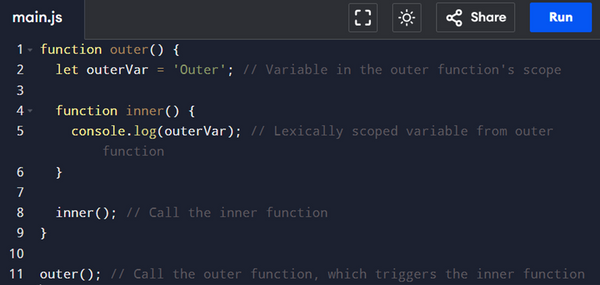
This sample code shows ‘outerVar’ is lexically scoped, meaning it is accessible inside the `inner` function because the `inner` function is declared inside ‘outer’.
Sample Output:

Sample Code in our sample application:
-
Variables like currentMonth, eventText, and functions like prevMonth are scoped within the component. They can only access variables and functions defined within the same component or its parent scope.
-
currentMonth` is scoped to the CalendarApp function.

Declaration Order of React.js:
In React.js, the declaration order refers to the sequence in which variables, constants, functions, hooks, and components are declared within a file or component.
Sequence of the Declaration Order in Our Sample Application:
1. Import Statements:
-
Modules and libraries should be imported first to clarify dependencies.

This code is importing the useState hook from React.
2. React Component Definition:
-
Defining the main functional component.

This function defines the component's structure and behavior and wraps all the logic that follows.
3. Constant Variables and Arrays (inside the component):
-
Keep static values and configurations at the top for easy reference and maintenance.

This code is declaring constants and arrays which are local to our component and are set up before the useState hooks because they are simple values that don’t need dynamic updates.
4. State Hooks (useState):
-
To manage dynamic data in the component (e.g., the current month, events, selected date).
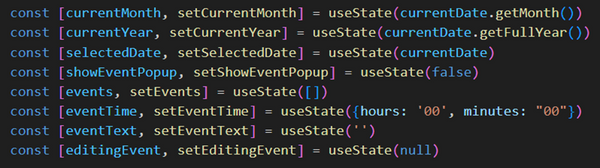
***State hooks must appear in a consistent order at the top of the function, because React relies on this order to keep track of state changes.
5. Computed Values:
-
Declaring computed values that depend on the state.

This part of code is based on the state hooks declared above and update whenever state changes.
6. Helper Functions:
-
Define reusable utility functions above the component body.
-
The helper functions in our sample application code are used to handle logic such as switching months, handling user input, or managing events. These functions are declared after the state hooks and computed values because they depend on that state:
-
prevMonth: Handles navigating to the previous month.
-
nextMonth: Handles navigating to the next month.
-
handleDayClick: Handles day selection and event popup display.
-
isSameDay: Checks if two dates are the same.
-
handleEventSubmit: Handles submitting or updating an event.
-
handleEditEvent: Opens an existing event for editing.
-
handleDeleteEvent: Deletes an event.
-
handleTimeChange: Handles changes in event time input.
7. JSX Return Block:
-
The JSX code is the component's UI structure, which uses the declared state, functions, and computed values

This code in our sample application is rendered based on the logic and data defined earlier in the component.
8. Export Statement:
-
Export statements appear last since they make the component available for other modules after it has been fully defined.

Finally, you export the component so it can be used in other parts of your application.
Reference Environment
The reference environment in React.js is influenced by JavaScript's scoping rules, the React component lifecycle, and the module system, which is shaped by JavaScript’s static scoping and augmented by React’s:
-
Component environment
-
State (declared with useState)
-
Props (passed form parent components)
-
-
Props
-
Context API
-
Closures (event handlers)
-
Module imports and exports
Named Constant in React.js:
React.js supports a variety of manifest (static) constants based on JavaScript's capabilities. The value of the constant is defined at compile-time or initialization and does not change throughout the application’s runtime.
Named constants in React.js are directly related to variable scope by determining where a named constant can be accessed and when it is created and destroyed. However, declaring named constants globally is not recommended, as they might cause naming conflicts and maintenance issues.