
Others
1. Multidimensional Array
​
A multidimensional array in JavaScript refers to an array that holds other arrays as its elements. This structure is commonly used to represent data in a grid or matrix form. Although JavaScript doesn't provide a specific syntax for multidimensional arrays, this can be accomplished by nesting arrays inside one another. (GeeksforGeeks, 2024).
Example: Creating a 2D Array
This code defines a 2D array mat in JavaScript, which is essentially an array of arrays. It then uses console.log to output the first inner array (mat[0]) and the value at the second row, third column (mat[1][2]), producing [1, 2, 3] and 6 as the output respectively.
​
​
​
Output:
​
​​​
​​
​​​​​​​​​
Methods and Techniques for Working with Multidimensional Arrays
-
Accessing Elements - Index-based element access.
-
Iterating Over Elements - Using loops (e.g., for loop or forEach).
-
Adding Elements - Methods like push(), unshift(), or direct assignment.
-
Removing Elements - Methods like pop(), splice(), shift(), or using delete.​
​​
2. Calling Subprograms Indirectly
​
There are situations in which subprograms must be called indirectly. These mostly occur when the specific subprogram to be called is not known until run time. The call to the subprogram is made through a pointer or reference to the subprogram, which has been set during execution before the call is made.
There are two common application types which uses indirect subprogram call:
-
Event Handling in Graphical User interface Applications
-
Callbacks when a subprogram is called and instructed to notify the caller when the its work is completed.



Sample Code in our sample application

Callback functions like prevMonth in this code are passed as handlers for events like onClick in React. They allow the program to indirectly invoke a subprogram (or function) in response to user actions such as clicking a button.​ This mechanism of passing a function as a reference (callback) to be executed later fits the concept of calling subprograms indirectly, where the function execution is deferred until the event is triggered.
3. Overloaded Subprograms (Function Overloading & Operator overloading)
​​
Function Overloading
Function Overloading is a feature found in many object-oriented programming languages, where multiple functions can share the same name but differ in the number or type of parameters. While languages like C++ and Java natively support function overloading, JavaScript does not support this feature directly.
​
In JavaScript, if two or more functions share the same name, the last defined function will overwrite the previous ones. This is because JavaScript treats functions as objects, and a subsequent function with the same name simply reassigns the function reference (GeeksforGeeks, 2024a).
​
Example Code in JavaScript:
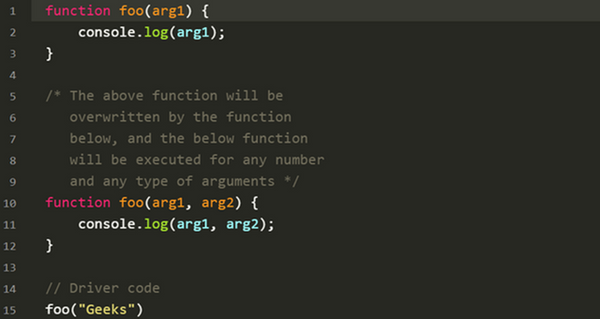
Based on the code provided above, the second function foo(arg1, arg2) overwrites the first function foo(arg1). When calling foo(“Geeks”), the function with two parameters is called, but the second argument remains undefined because only one argument was passed.
Output:

​
Operator Overloading
Operator overloading is the ability to redefine the behavior of operators (e.g., +, -, *, etc.) for user-defined types. This is supported in languages like C++ and Python.
However, JavaScript does not support operator overloading. The behavior of operators is fixed and cannot be redefined for custom objects or types.
4. Setting State
​
setState is a method used in React class components to update the component’s state that triggers a re-render with the updated values. All the React components can have a state associated with them. The state of a component can change either due to a response to an action performed by the user or an event triggered by the system.
​​
Besides that, setState in React is an asynchronous call means if a synchronous call gets called it may not get updated at the right time like to know the current value of an object after an update using setState it may not give the updated value on the console.
​
We use the setState() method to change the state object. It ensures that the component has been updated and calls for re-rendering of the component (GeeksforGeeks, 2024b).
Sample Code in our sample application
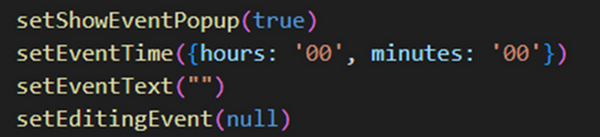
These functions demonstrates state management in React using useState hooks to handle date comparison and event submission logic. The code also includes state-setting functions which are part of the React component’s state management.
​
Each setState function is responsible for managing a specific part of the component's state:
-
setShowEventPopup: Controls the visibility of the event popup.
-
setEventTime: Initializes or resets the event time.
-
setEventText: Clears or resets the event description field.
-
setEditingEvent: Manages whether the user is editing an existing event or creating a new one.
This aligns with React's core philosophy, where state updates trigger re-renders to ensure the UI stays in sync with the application’s data. The code highlights the importance of state management for interactive and dynamic applications, such as resetting inputs or toggling components like event popups.