
Semantics Models for
Parameter Passing
1. In Mode (Pass-by-Value)
-
Characteristics:
-
Receives data from the corresponding actual parameter.
-
The value of the actual parameter is used to initialize the formal parameter.
-
Typically implemented by copying.
-
-
Implementation Options:
-
Copying:
-
Efficient but requires additional storage (stored twice).
-
Copying large parameters can be costly.
-
-
Access Path (not recommended):
-
Requires write protection, which is hard to enforce.
-
Indirect addressing increases access costs.
-
-
-
In JavaScript:
-
Primitive types (numbers, strings, booleans, etc.) are passed by value.
-
The formal parameter in the function is a copy of the actual parameter, so modifying it does not affect the original.
Example in JavaScript:
Output:
-
2. Out Mode (Pass-by-Result)
-
Characteristics:
-
Transmits data to the actual parameter.
-
No value is initially transmitted to the subprogram; the formal parameter acts as a local variable.
-
Value is transmitted back to the caller’s actual parameter when control is returned.
-
-
Disadvantages:
-
Requires extra storage and copy operations (for physical moves).
-
-
In JavaScript:
-
JavaScript does not directly implement pass-by-result. Functions in JavaScript return values explicitly, rather than modifying parameters after the function ends.
-
To simulate this behavior, you would return a value from the function and assign it to the caller.
-
3. Inout Mode (Pass-by-Value-Result or Pass-by-Reference)
-
Characteristics:
-
Can transmit and receive data (combines in and out mode).
-
Known as pass-by-copy or pass-by-value-result.
-
Local storage is used for formal parameters.
-
-
Pass-by-Reference (Pass-by-Sharing):
-
Passes an access path (pointer/reference).
-
Efficient passing process.
-
-
Disadvantages:
-
Slower access compared to pass-by-value.
-
Potential for unwanted side effects and aliases.
-
-
In JavaScript:
-
Pass-By-Value-Result is not directly implemented in JavaScript, but similar behavior can be mimicked with functions that return values.
-
In this approach, a copy of the value is passed to the function. The function works with this copy, and after the function finishes executing, the final result can be assigned back to the original variable (if explicitly handled by the programmer).
-
4. Parameter Passing in React.js
-
In Mode and Inout Mode:
-
Parameters (arguments) are passed by value in React.js.
-
The function only receives values, not the locations of the arguments.
-
Updates made to arguments do not affect the original values after the function call.
-
-
Objects in JavaScript:
-
In JavaScript, object references are values.
-
Because of this, objects will behave like they are passed by reference:
-
If a function changes an object's property, it changes the original value.
-
Changes to object properties are visible (reflected) outside the function (W3Schools.com, n.d.-d).
-
-
No Pointers in JavaScript (Unlike C++):
-
Objects are passed by reference but the reference itself cannot be manipulated.
-
The programmer works with a copy of the reference, not the actual memory address of the object (Are There Pointers in Javascript?, n.d.).
-
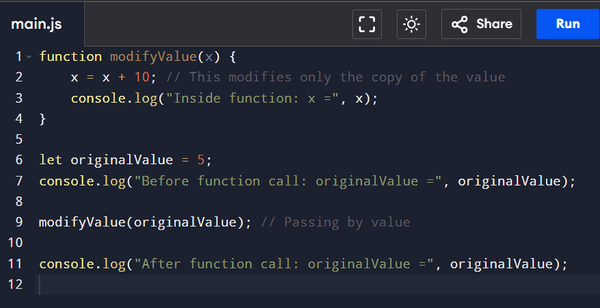
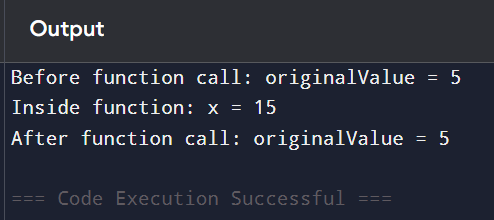
Sample Code in our sample application
