Type Conversation
Narrowing Conversion
Narrowing Conversion is one that converts an object to a type that cannot include all the values of the original type. In React.js, narrowing conversions refers to transforming the type of a variable in a conditional block by the means of runtime tests. This is done with help of such methods as typeof checks, instanceof checks or checks for the property’s existence to provide better typing in consequent code execution. Coercion type guards in React.js use typeof to identify one type among a set of primitive types. For instance, the code check, if typeof variable === ‘string’ will help you reduce the type of the variable to string only within that block of code (GeeksforGeeks, 2024).
Example: The function myFunction takes a parameter value of type string or number. If the value is a string, it's converted to uppercase; if it's a number, it's formatted to 2 decimal places using toFixed().
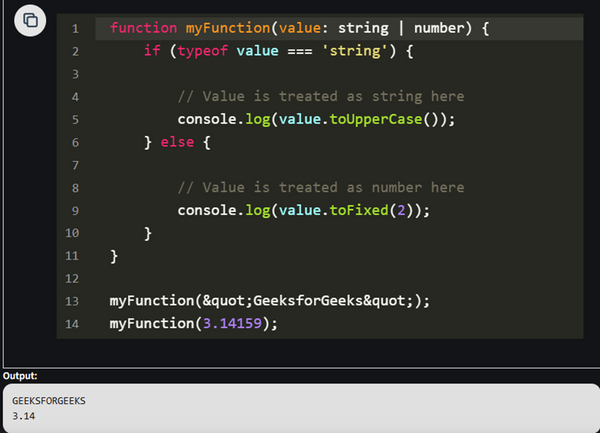
In React.js the in operator checks whether a particular property exists in an object. In type guards, it distinguishes types based property presence ‘ ‘. For example, when we have property in an object, then the type is restricted to that property.
Example: The function area calculates the area of a square or rectangle. It uses the in operator to distinguish between them based on property existence. If shape has sideLength, it computes the square area; otherwise, it calculates the rectangle area.
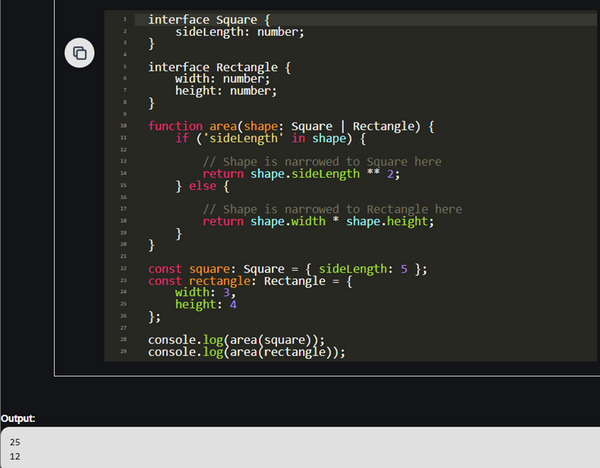
Sample Code for React.js in our sample application:
-
Date.now() generates a timestamp as a number, which is assigned to the id property of the event.

Widening Conversion
A widening conversion is one in which a fraction converts to more than, but fewer than another fraction object is transformed into a type of data are likely to contain at least approximations of all the values of the original type. A widening conversion in JavaScript happens when we transfer a value of a different narrower type, such as a number, boolean or null, to a broader type, such as string or object. For example, it may be possible to widen the value because string can contain much more content than a number. It would be called as a widening conversion, cos an object can contain things that a boolean cannot contain. In React.js, four broad classes of widening conversions are possible, when data is passed through props or states, where wider types are converted from some tighter types to accommodate differing and potentially more richer parameters (W3Schools.com, n.d.-c).
Sample Code for React.js in our sample application:
-
The eventTime.hours and eventTime.minutes values are numbers but are automatically converted to strings because of template literals (${}).
-
This is an example of implicit type conversion (widening) since numbers are converted into strings to form the time string.

Mixed Mode Expression
A mixed-mode expression is one that has operands of different types. A coercion is an implicit type conversion by compiler. The disadvantages of coercions, they decrease in the type error detection ability of the compiler (W3Schools.com, n.d.-c).
Sample Code for React.js in our sample application:
-
The eventTime.hours and eventTime.minutes (both strings) are combined into a single string using template literals (${}), along with the ":" character.
-
If there were a direct concatenation between numbers and strings in your code, it would implicitly convert the number to a string during the operation.

Explicit Type Conversions
In React.js, perhaps you find it necessary to force the type of the data that is being passed to other components or to be rendered in JSX. Coercion involves the conversion of one data type to another one; by specifically converting the documents to the expected type of data, you avoid the pitfall that come with coercion. This is particularly important when working with different basics data time in the JSX structure, handling forms, or as an argument for functions between composed components in React.js(W3Schools.com, n.d.-c).
Some examples include:
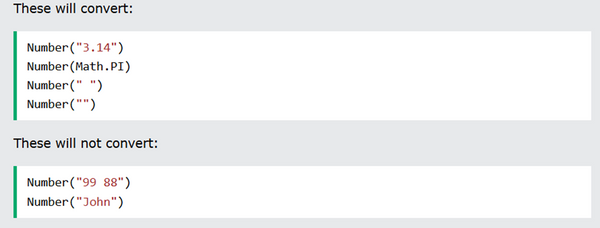
-
The global method Number() converts a variable (or a value) into a number.
-
A numeric string (like "3.14") converts to a number (like 3.14).
-
An empty string (like "") converts to 0.
-
A non-numeric string (like "John") converts to NaN (Not a Number).
The unary + operator can be used to convert a variable to a number:

If the variable cannot be converted, it will still become a number, but with the value NaN (Not a Number):

The global method Number( ) can be used to convert dates to numbers.

The date method getTime( ) does the same.

Errors in Expressions
In React.js, these errors are basically caused by the inherent limitations of arithmetic for example division by zero. Limitations of computer arithmetic, for example overflow, result of an operation cannot be represented in the memory cell where it must be stored. This is always ignored by the run-time system which is called run-time errors (Understanding Errors in JavaScript | Web Reference, n.d.).
​
JavaScript errors can happen for a variety of reasons, some of which include:
-
How to write code with incorrect syntax?
-
Using undefined variables
-
Interacting with objects in a program namely applying functions on them if not functions
-
Supposing values that are invalid when they were meant to be numbers
-
Casting a value beyond its permitted zone
-
Unknown object property or maybe serve as a joke
-
Trying to run code which has been prevented from running due to the browser’s security settings
-
Communicating with third-party libraries or APIs that have defects or with which a developer does not want to deal
Such situations can arise by code errors, pitfalls in other libraries, or user inputs and different conditions. Depending on such a plan it is recommendable to make preparations for errors that may occur and how to deal with them. If they are familiar with the causes, then they will not allow such incidences to occur and write better code.
​​
Syntax errors are those which include written code not obeying the rules of writing for JavaScript language. This can comprise of missing semicolons or incorrect use of quotes, or when we have parentheses that do not match. In fact these are simple to identify and correct because whenever the JavaScript interpreter chokes on the code it dictates the line containing the error.
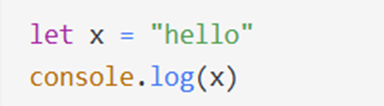
In the above example if we omit semicolon at end of the first line it will through an error.
Runtime errors that involve reference are committed when the reference is made on a variable or an object, which has not been declared. Often this occurs, when you typed a variable name incorrectly or you did not use var, let, const and similar statements for the variable declaration.

Parameter y is assigned empty before it is invoked, which results into reference error.
Type errors are the kind of error that occur when the code is of certain data type while the program expected the data to be another type. This can be because of attempting to perform a method on a non-object value, or as a result of trying to access attributes of an undefined object.
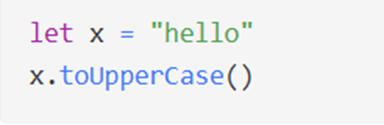
In the above example, the variable x is a string, but we are trying to invoke the toUpperCase() method on it, which can only be invoked on String objects so it will be a type error.
​
Range errors arise when a particular value is out of range and deviates from the normal average. This can occur for example when someone attempts to create an array whose size is a negative integer value or when someone tries to apply index number that is greater than or equal to the size of the array to an array.

In the above example, we’re interested in setting up the array and as you can see, we are passing negative value for the length which results in a range error.
Sample Code for React.js in our sample application:
-
Errors in expressions can occur due to invalid operations or type mismatches. The provided code includes a check to prevent errors by limiting the length of the event text.
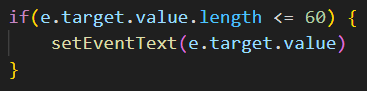